Looking to add an array to your BASH script? In this tutorial we will cover everything you need to know, and perhaps a few extra things that will surprise you!
BASH scripts are a powerful feature of POSIX based operating systems, giving the user the power to automate many operating system features with a relatively easy to learn programming language.
Arrays are a staple of almost every programming language and BASH script is no exception.
This tutorial will teach you about the types of arrays that we can use in BASH script as well as giving examples on how to use them.
- Prerequisite
- What is an array in BASH?
- How to create an array in BASH script
- Associative array
- Indexed array
- Adding elements to the array
- Echo the values in the array
- Get the size of the array
- Get the size of an element in the array
- Copying the array
- Adding new items to the array
- Listing elements from an indexed position
- Extracting parts of a value within the array
- Find and replace array items
- Removing items from the array
- Removing items using a wildcard
- Conclusion
Prerequisite
I will assume that as you have arrived here, you are already running some flavour of Linux or POSIX compatible operating system.
Mac OS X users can also make use of this BASH scripting tutorial!
In case you are looking for a platform, the Raspberry Pi is a great little device to hone your Linux skills and avoids having to dual boot your main computer.
If you are a completed beginner then no need to worry!
This tutorial is very simple and should be easy enough to follow for someone who has managed to get to the Linux command prompt in one way or another.
If you have already written some simple BASH scripts then feel free to skim over the introduction.
What is an array in BASH?
An array in BASH is like an array in any other programming language.
An array is a variable that can hold multiple values, where each value has a reference index known as a key.
In BASH script it is possible to create type types of array, an indexed array or associative array.
It is also worth noting that one limitation of a BASH arrays is that you cannot create a multidimensional array, such as placing an array within an array.
Associative array
In an associative array the key is written as a string, therefore we can associate additional information with each entry in the array.
The disadvantage is we cannot perform arithmetic operations on the key, but more on that later.
MyFoodArray | Key |
Toast | breakfast |
Sandwich | lunch |
Pizza | dinner |
Indexed array
In an indexed array the key is a numerical value, which increments upwards for each value within the array. We can represent a simple array using a table for better visualisation.
MyFoodArray | Key |
Toast | 0 |
Sandwich | 1 |
Pizza | 2 |
How to create an array in BASH script
So now that we understand the basic principles of the two types of array that we can create with BASH script, let’s take a look at some examples of how we can use this within our script.
Associative array
First let’s take a look at an associative array. Rather than using numerical values, we can use strings that represent the index of the value. In order to create an associative array, we must declare it using the “-A’ flag.
Adding elements to the array
Using our example of food from the table above, let’s go ahead and create an associative array. We can specify the association within [square brackets] and then equal it to the value.
#!/bin/bash declare -A MyFoodArray MyFoodArray[breakfast]="toast" MyFoodArray[lunch]="sandwich" MyFoodArray[dinner]="pizza"
We can simplify this down to a single line by specifying the entries into the array at the same time as the declaration. Each association is still given in [square brackets] and then made to equal to the value.
#!/bin/bash declare -A MyFoodArray=([breakfast]="toast" [lunch]="sandwich" [dinner]="pizza")
Echo the values in the array
Now that we have created an array and loaded some values into it, let’s look at how we can use it. A simple example would be to echo the contents of the array in the terminal.
We can choose the item from the array that we wish to print by referencing it with the name of the association. For example to return the value “toast” we can echo the value stored in “breakfast.”
#!/bin/bash declare -A MyFoodArray=([breakfast]="toast" [lunch]="sandwich" [dinner]="pizza") echo ${MyFoodArray[breakfast]}
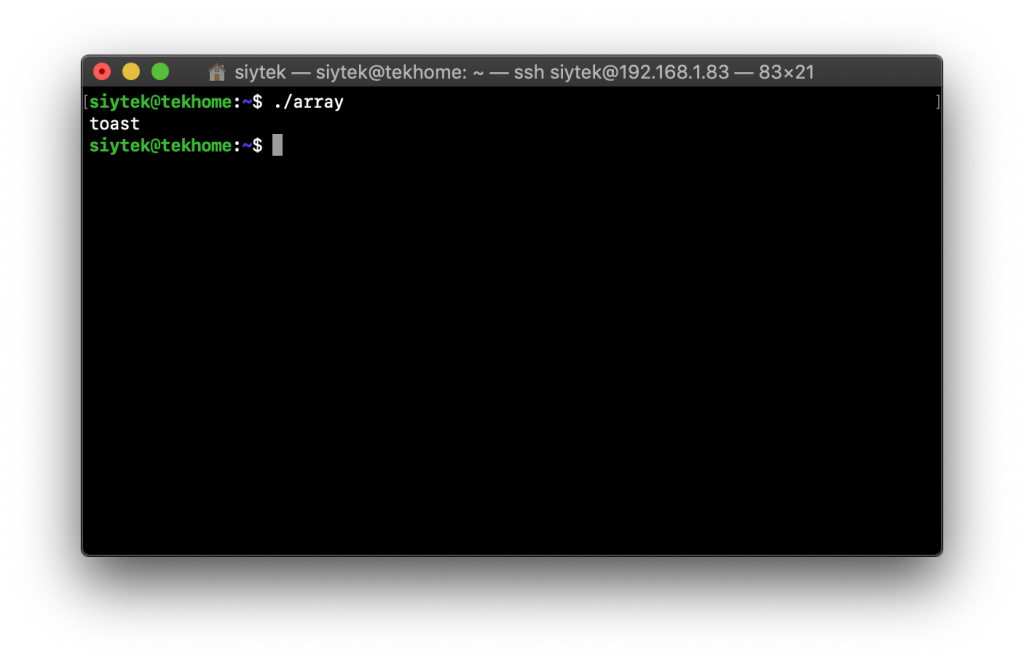
If we wish to echo the entire contents of the array, we can specify ‘@’ for the key. This will echo the entire contents of the array in the terminal.
#!/bin/bash declare -A MyFoodArray=([breakfast]="toast" [lunch]="sandwich" [dinner]="pizza") echo ${MyFoodArray[@]}
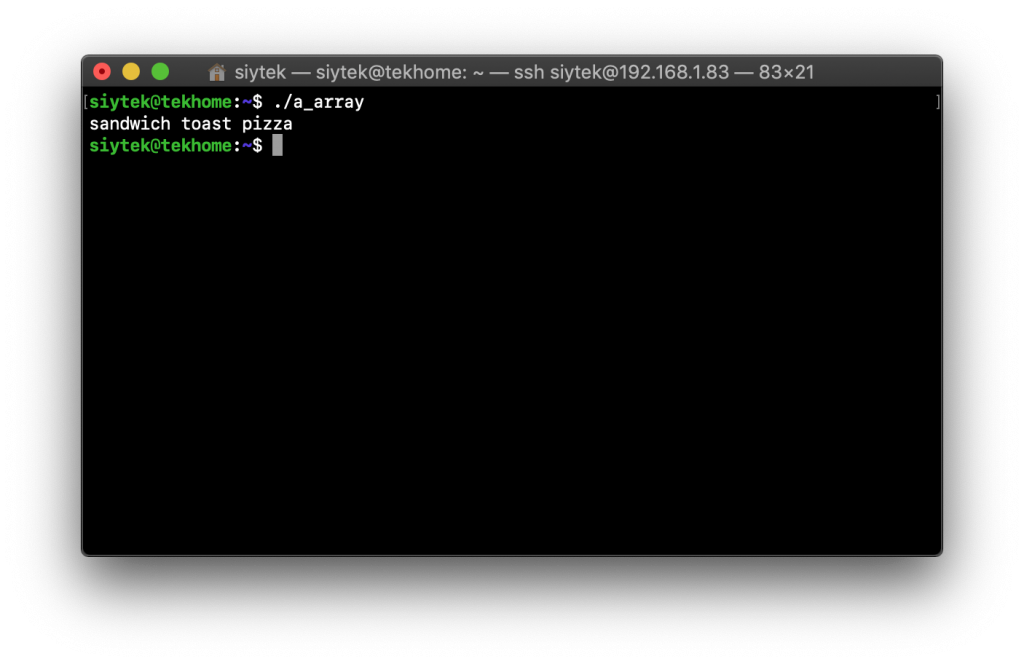
Echo the keys in the array
Sometimes it may be necessary to access the names of the keys within the array. In order to do this we can use the “!” operator before the name of the array.
#!/bin/bash declare -A MyFoodArray=([breakfast]="toast" [lunch]="sandwich" [dinner]="pizza") echo ${!MyFoodArray[@]}
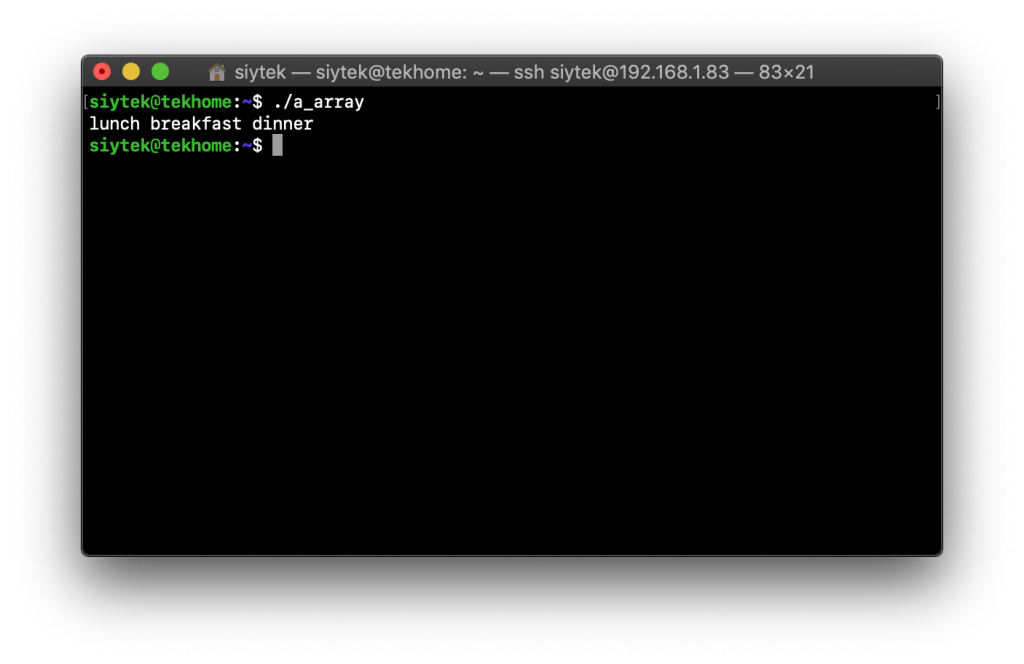
Adding new items to the array
We can add a new association to the array simply by using the “+=” operator. The new key can be specified with [square brackets] and made equal to a new desired value.
#!/bin/bash declare -A MyFoodArray=([breakfast]="toast" [lunch]="sandwich" [dinner]="pizza") MyFoodArray+=([desert]="icecream") echo ${!MyFoodArray[@]}
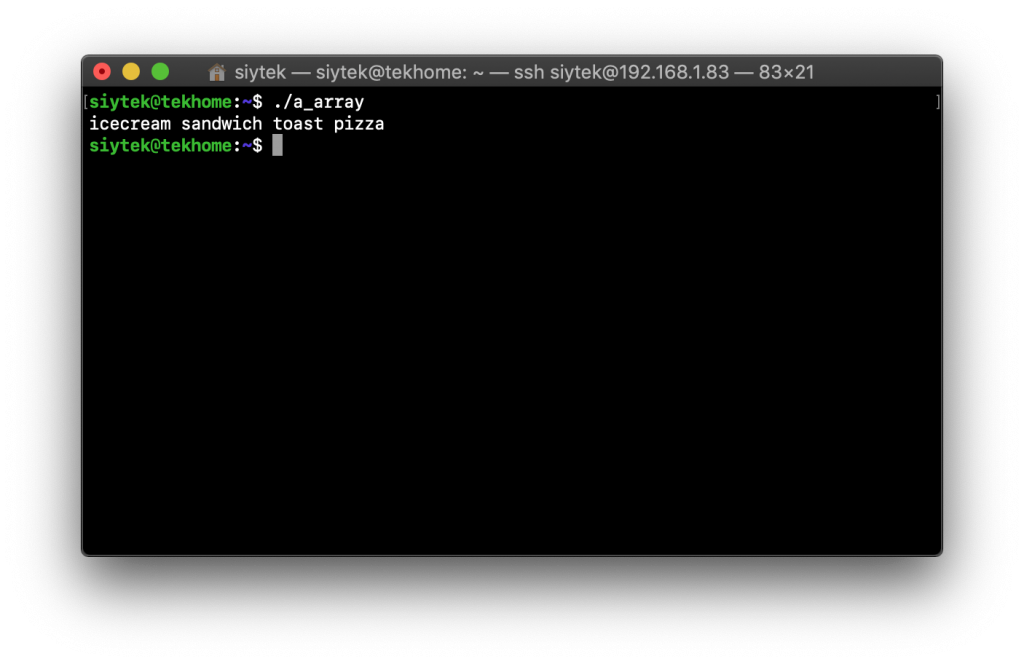
Deleting items from the array
It is very straightforward to remove an item from the array simply by using the unset command.
#!/bin/bash declare -A MyFoodArray=([breakfast]="toast" [lunch]="sandwich" [dinner]="pizza") unset MyFoodArray[dinner] echo ${!MyFoodArray[@]}
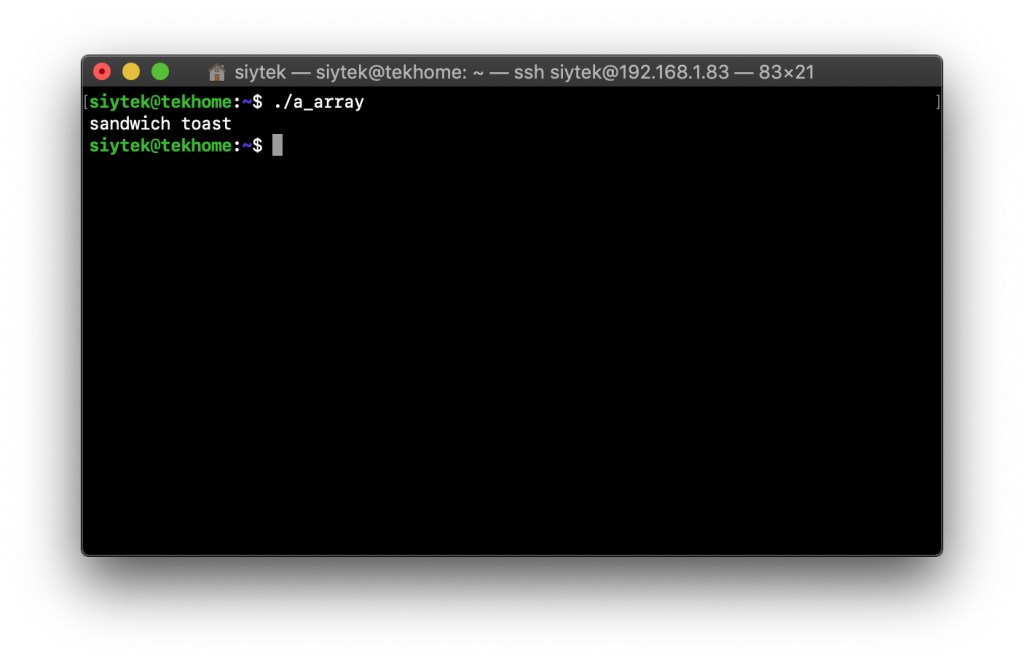
Indexed array
Now let’s take a look at the more commonly used indexed array. There are several ways that we can create an array and add elements to it.
Adding elements to the array
Using our example of food from the table above, let’s go ahead and create an indexed array. Each element will be placed into the indexed location specified within the [square brackets].
#!/bin/bash MyFoodArray[0]="toast" MyFoodArray[1]="sandwich" MyFoodArray[2]="pizza"
We can simplify this to a single line by declaring the array and all of the values we wish to assign. As this is a numerically indexed array, we will declare it with the “-a” flag. Each entry into the array is simply separated by a space and will be loaded into the index sequentially.
#!/bin/bash declare -a MyFoodArray=("toast" "sandwich" "pizza")
Echo the values in the array
Now that we have created an array and loaded some values into it, let’s look at how we can use it. A simple example would be to echo the contents of the array in the terminal.
We can choose the item from the array that we wish to print by referencing it with the associated index value. This will echo the value stored in the array at position [0].
#!/bin/bash declare -a MyFoodArray=("toast" "sandwich" "pizza") echo ${MyFoodArray[0]}
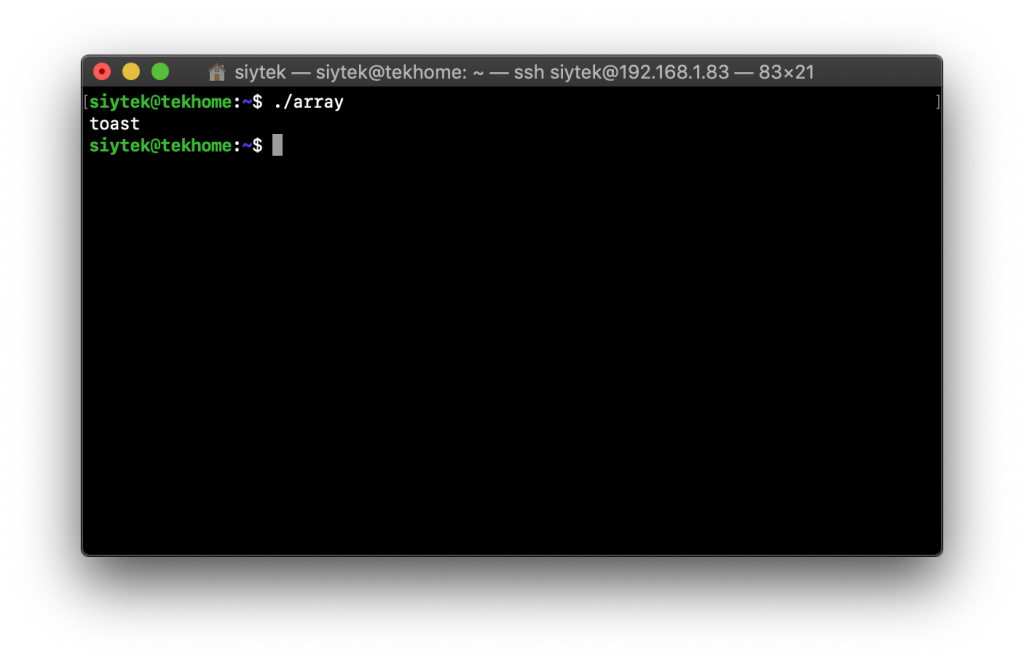
If we wish to echo the entire contents of the array, we can specify ‘@’ for the key. This will echo the entire contents of the array in the terminal.
#!/bin/bash declare -a MyFoodArray=("toast" "sandwich" "pizza") echo ${MyFoodArray[@]}
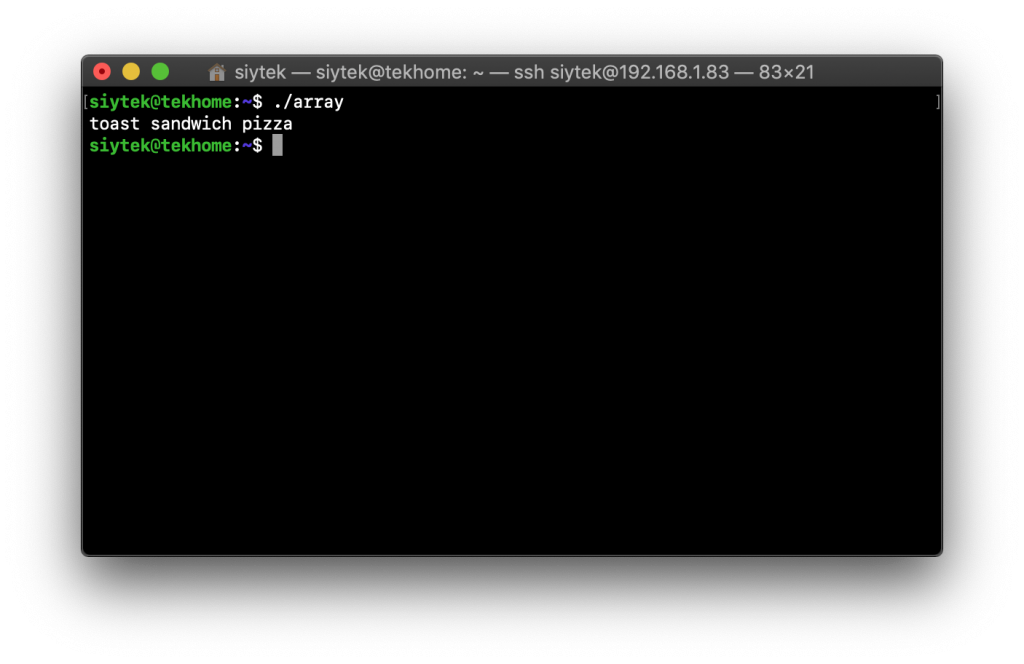
Get the size of the array
It is also possible to get the number of elements stored in an array. This can be useful if we need to write some code that can find the end of the array. This is done simply by adding a ‘#’ symbol to the beginning of the array name.
#!/bin/bash declare -a MyFoodArray=("toast" "sandwich" "pizza") echo ${#MyFoodArray[@]}
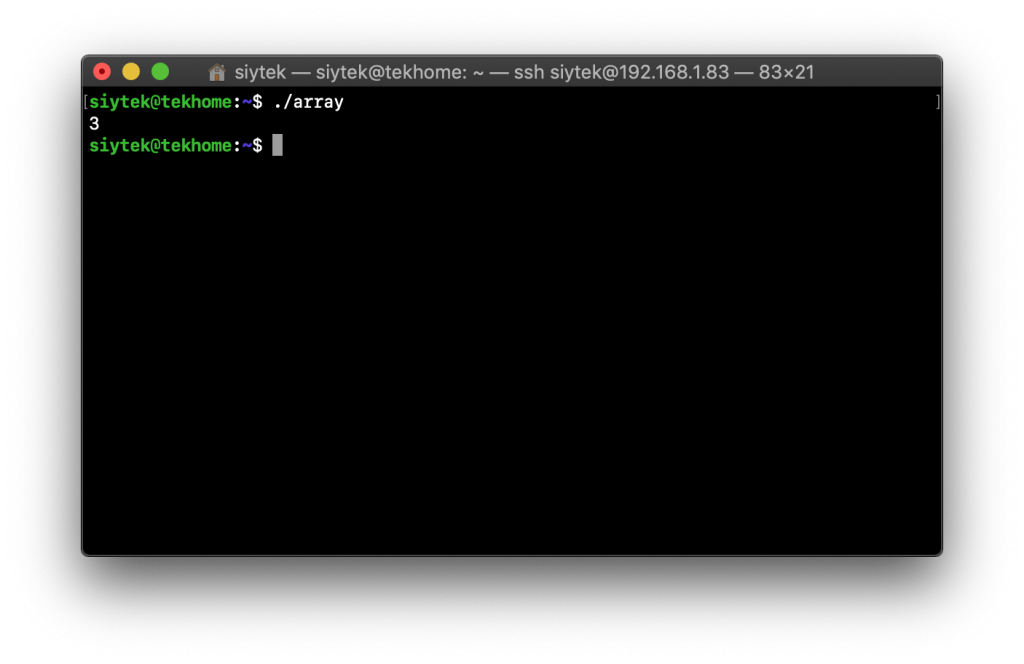
Get the size of an element in the array
We can also use the ‘#’ operator to get the size of individual elements inside of the array. For example the entry ‘sandwich’ indexed in position 1 has 8 characters.
#!/bin/bash declare -a MyFoodArray=("toast" "sandwich" "pizza") echo ${#MyFoodArray[1]}
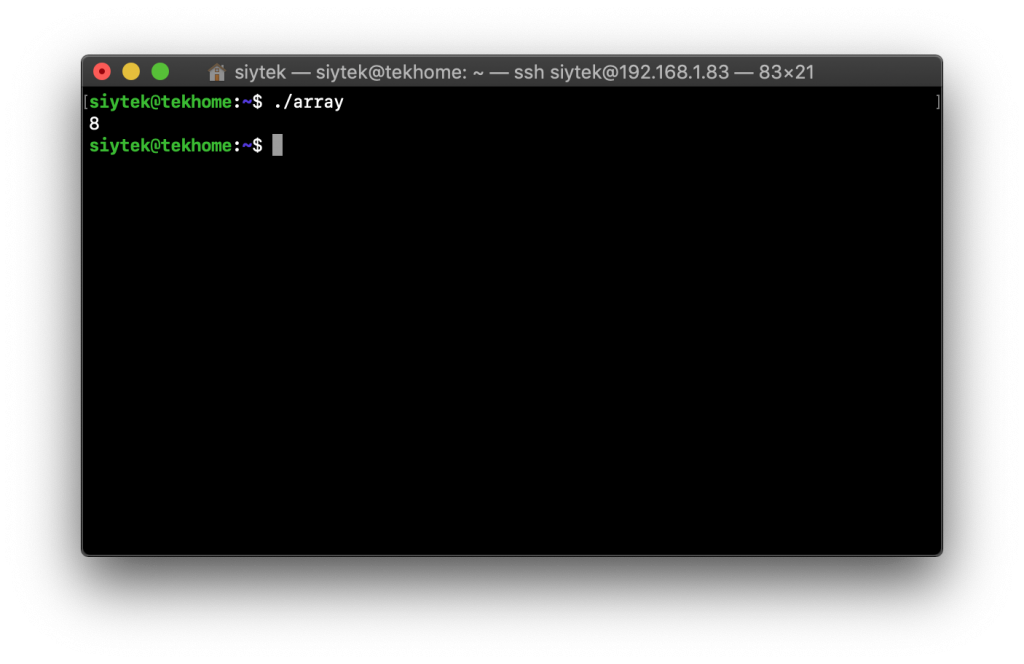
Copying the array
It is possible for us to copy an array simply by making the original array equal to the new array. We must use the “@” symbol for the index in order to copy all elements from the original array to the new array.
#!/bin/bash declare -a MyFoodArray=("toast" "sandwich" "pizza") AlsoMyFoodArray=(${MyFoodArray[@]}) echo ${AlsoMyFoodArray[@]}
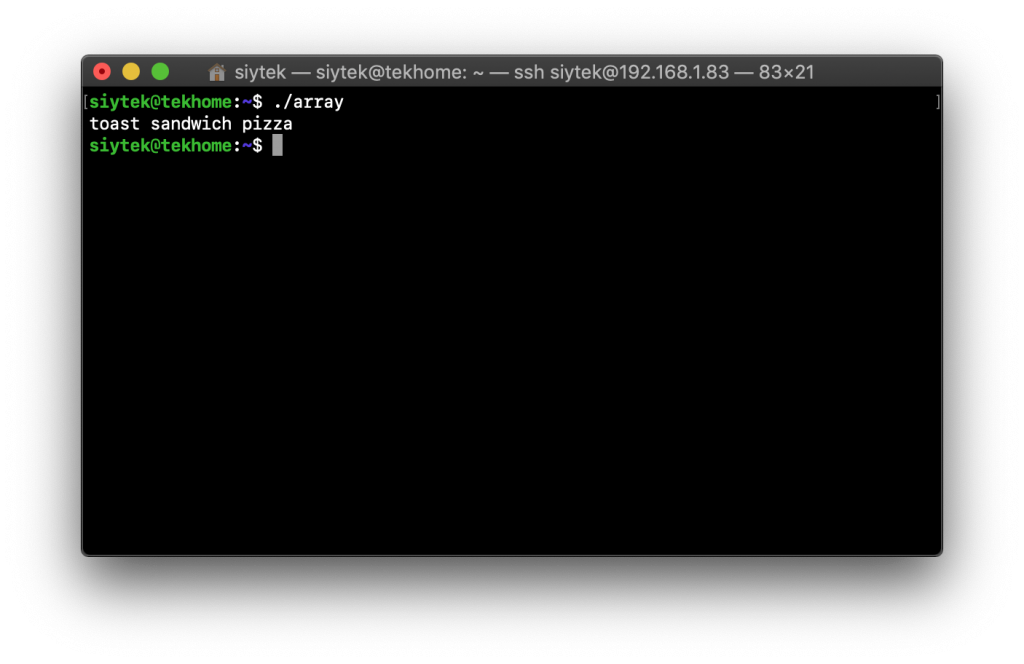
Adding new items to the array
In order to add new items to an array we can simply redefine the array as itself, plus the additional items that we want to add.
#!/bin/bash declare -a MyFoodArray=("toast" "sandwich" "pizza") MyFoodArray=(${MyFoodArray[@]} "chocolate" "icecream" "pasta") echo ${#MyFoodArray[@]}
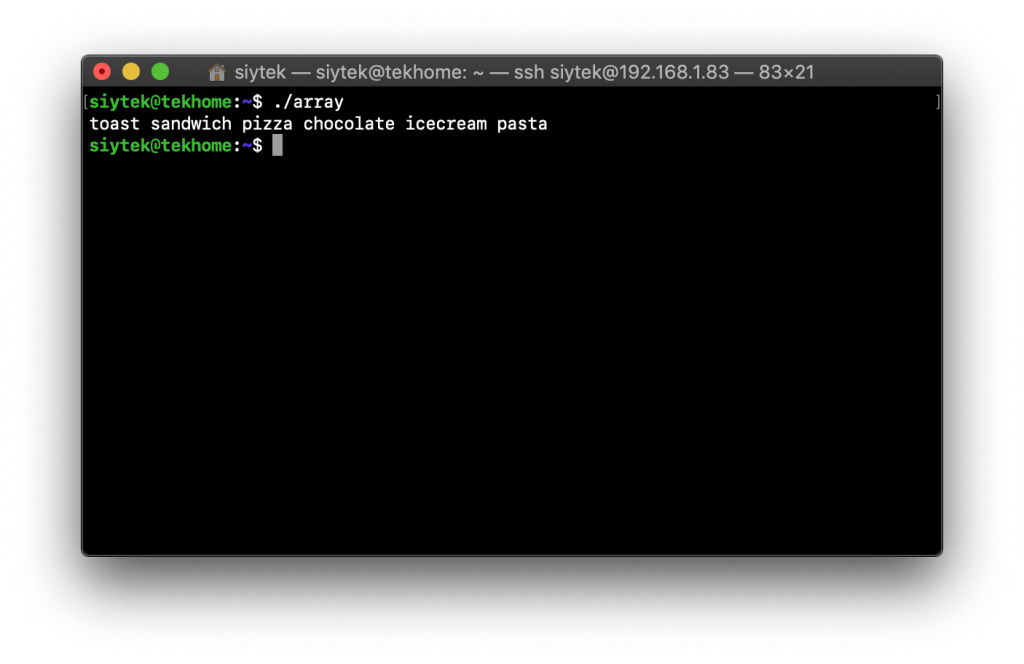
Listing elements from an indexed position
It is possible for us to specify a starting point within our array and then list any number of entries within the array sequentially.
For example we can specify index position 1 and then print this value and the following 3 values.
In order to do this we must specify the start point within the array and then the number values we wish to sequentially access.
#!/bin/bash declare -a MyFoodArray=("toast" "sandwich" "pizza" "chocolate" "icecream" "pasta") echo ${MyFoodArray[@]:2:4}
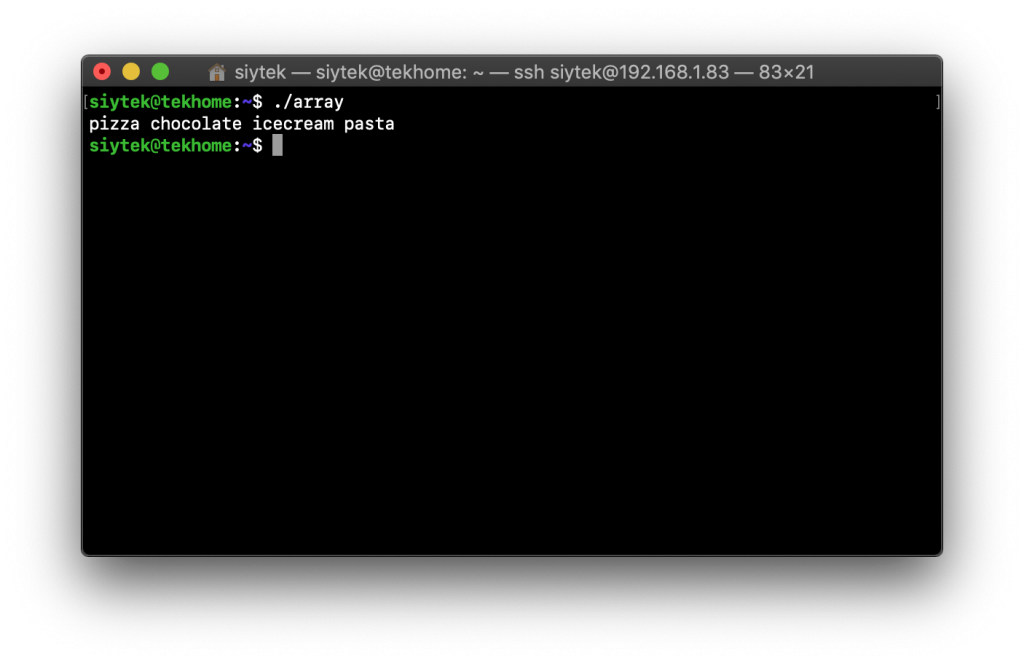
Extracting parts of a value within the array
We can use the same syntax as above with individual elements in the array. For example let’s say we want to extract the word ‘cream’ from ‘icecream’ which is indexed in the array with the key number 4.
We aim to extract from index [4] and we are interested in characters 3 through to 7, which is the word “cream.” Don’t forget the first letter ‘i’ will be referenced as 0 and not 1.
#!/bin/bash declare -a MyFoodArray=("toast" "sandwich" "pizza" "chocolate" "icecream" "pasta") echo ${MyFoodArray[4]:3:7}
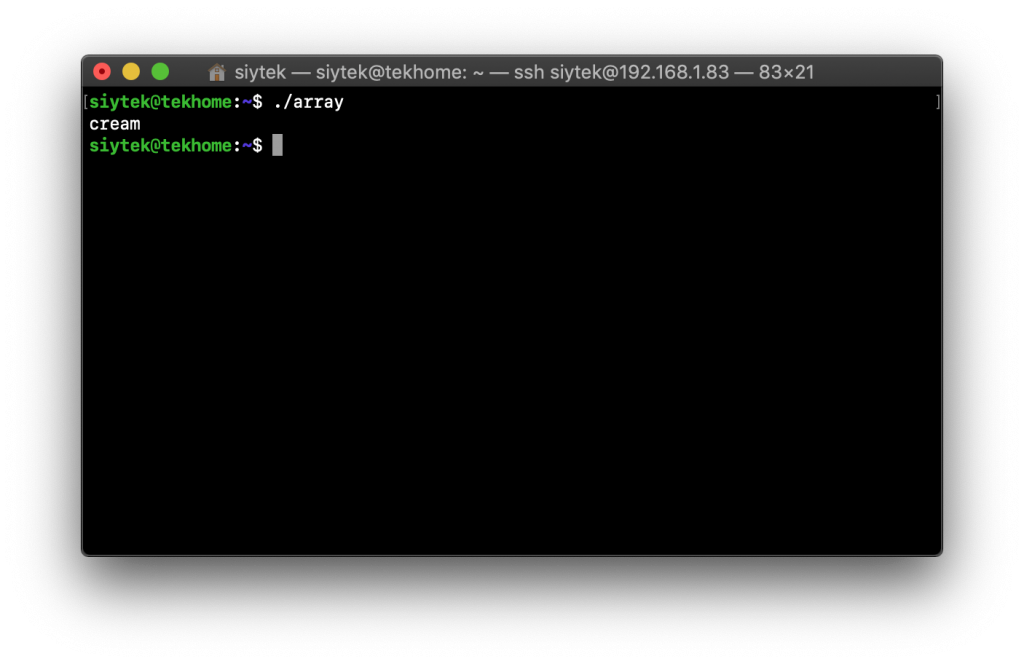
Find and replace array items
Another useful function that we can perform on an array is to find an entry within the array that matches a search term, and then replace it with something else.
For example we can replace the food item “pasta” with “jelly” using the following syntax.
#!/bin/bash declare -a MyFoodArray=("toast" "sandwich" "pizza" "chocolate" "icecream" "pasta") echo ${MyFoodArray[@]/pasta/jelly}
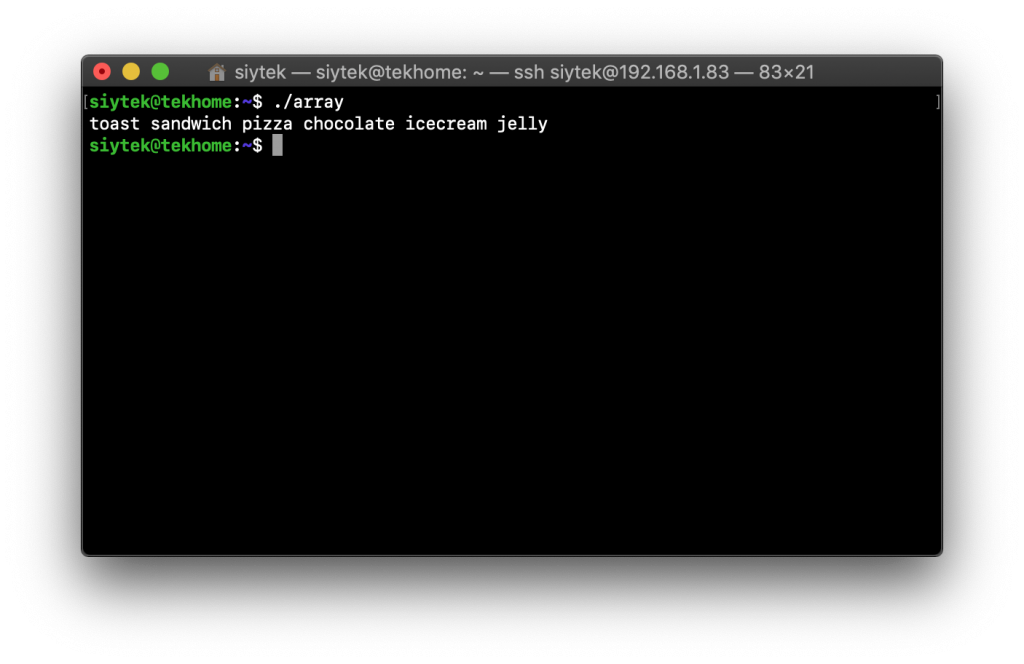
Removing items from the array
Removing items from an array can be a little more tricky and requires understanding from the previous examples, which is why I left it until a little later. It is easy to empty the contents stored at an indexed location using the unset comand.
#!/bin/bash declare -a MyFoodArray=("toast" "sandwich" "pizza" "chocolate" "icecream" "pasta") unset MyFoodArray[2] echo ${MyFoodArray[@]}
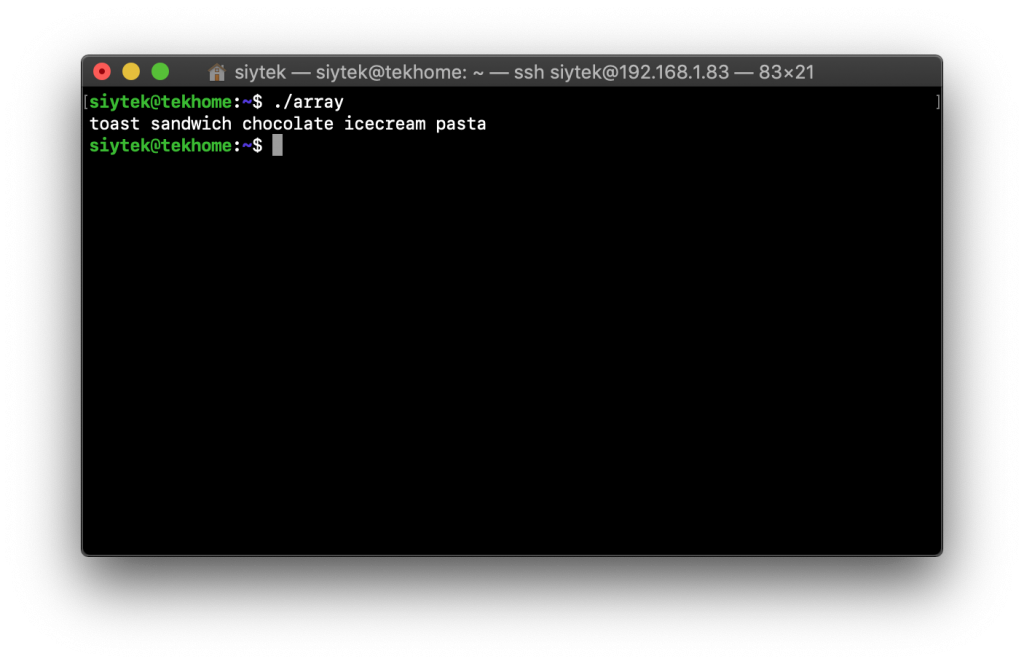
As you can see from the output this produces the expected results, “pizza” has been removed from the list. However we did not actually remove the entry from the array, we only gave it a null value. For example “chocolate” still remains at position 3.
#!/bin/bash declare -a MyFoodArray=("toast" "sandwich" "pizza" "chocolate" "icecream" "pasta") unset MyFoodArray[2] echo ${MyFoodArray[0]} echo ${MyFoodArray[1]} echo ${MyFoodArray[2]} echo ${MyFoodArray[3]} echo ${MyFoodArray[4]}
If we run the code above we can see that there is a blank space when we try to print position 2 and the end value has been truncated. This is because we only emptied the contents and did not actually remove it entirely.
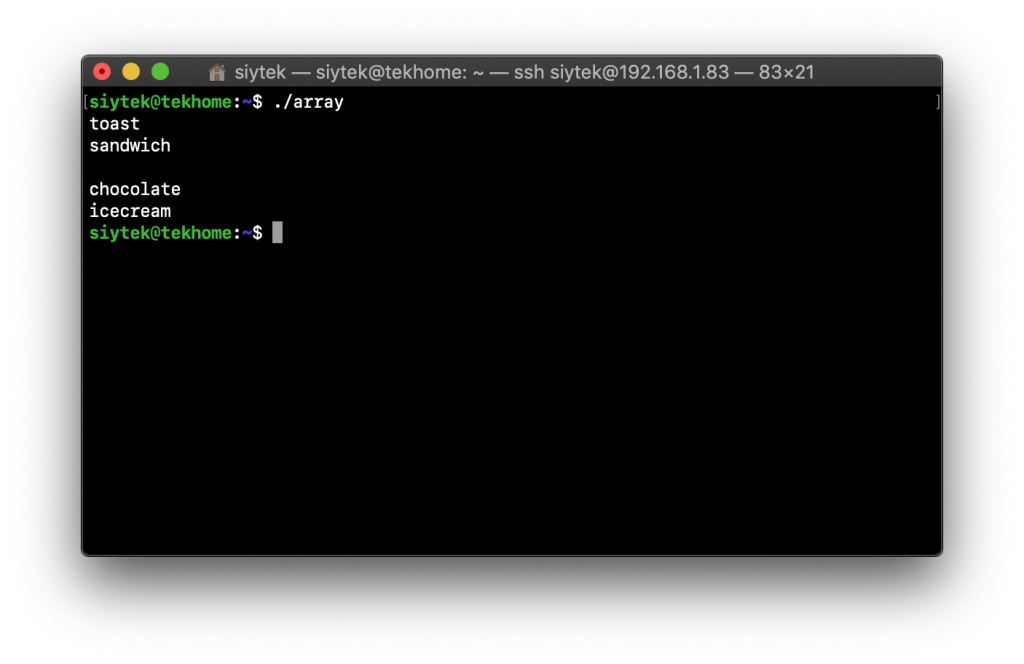
In order to completely remove the value from the array we need to renumber all of the entries following the one that we deleted. A simple way to do this is by setting the array value to itself, but skipping over the value we wish to remove.
#!/bin/bash declare -a MyFoodArray=("toast" "sandwich" "pizza" "chocolate" "icecream" "pasta") DeletePosition=2 MyFoodArray=("${MyFoodArray[@]:0:$DeletePosition}" "${MyFoodArray[@]:$(($DeletePosition + 1))}") echo ${MyFoodArray[0]} echo ${MyFoodArray[1]} echo ${MyFoodArray[2]} echo ${MyFoodArray[3]} echo ${MyFoodArray[4]}
Now we can see that all of the values that follow the deleted value have been moved backwards in the index in order to fill the gap.
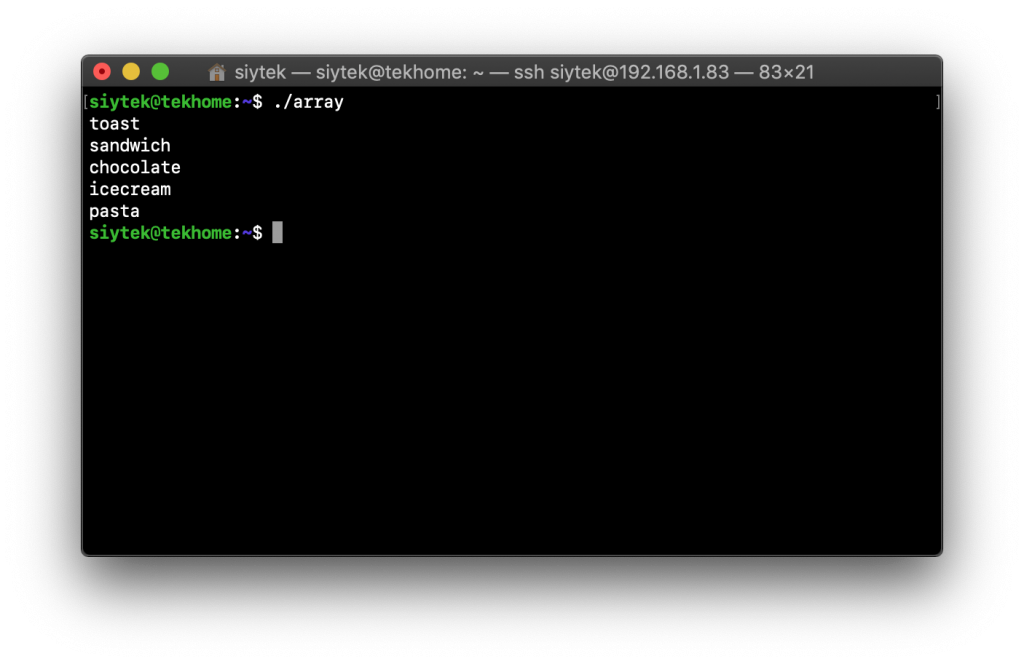
Removing items using a wildcard
We can also remove items using the wildcard character (*). In order to do this we will use the find and replace method from earlier, but we will set the value to null. Then we can load the results into a new array.
#!/bin/bash declare -a MyFoodArray=("toast" "sandwich" "pizza" "chocolate" "icecream" "pasta") declare -a FoodNotP=(${MyFoodArray[@]/p*/}) echo ${FoodNotP[@]} echo ${#FoodNotP[@]}
This script will take all of the items that do not begin with “p” and load them into a new array called FoodNotP. It will also print the length of the newly created array. We can see that there are no null values in the array where entries beginning with “p” have been removed.
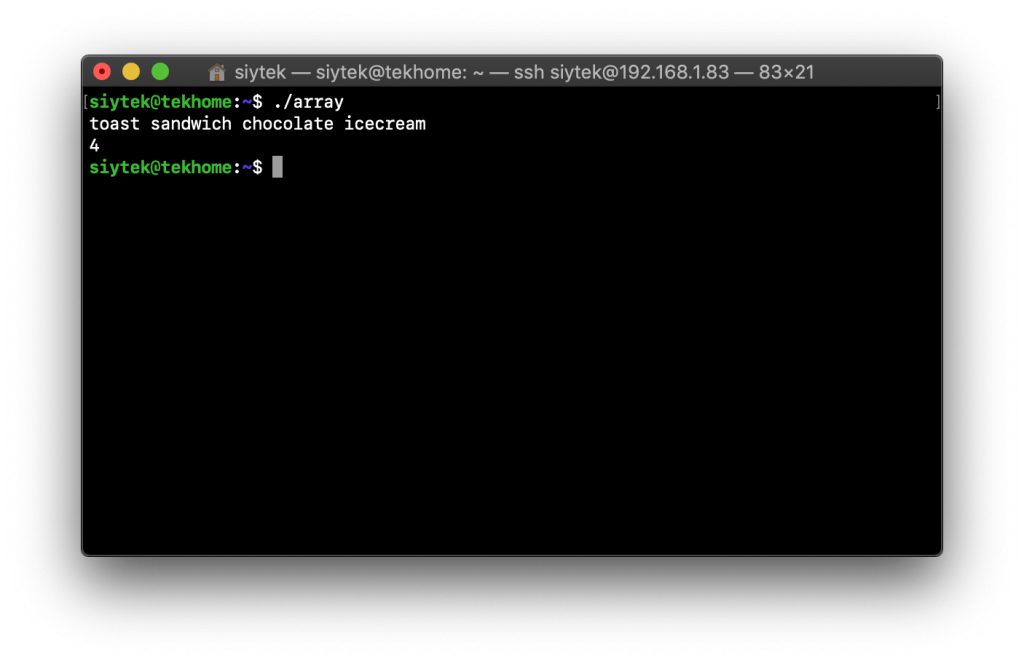
Conclusion
Finished, well done! Whilst your Linux skills are on fire, you should definitely check this out next!
Arrays are a stable part of mostly all programming languages and BASH scripting is no exception. In this tutorial we have covered many different examples of how to manipulate the data within an array.
You should now have enough understanding to apply this to your own BASH scripting projects!
For further reading you can go ahead and check out some more of my Linux-related tutorials. You can also apply these Linux tutorials to the fabulous Raspberry Pi platform!
Article Updates
Aug 19th 2022 : Minor formatting changes. Syntax highlighting plugin changed. Featured image updated. Fixed broken links in table of contents
Article first published April 20th 2020.
Thanks so much for visiting my site! If this article helped you achieve your goal and you want to say thanks, you can now support my work by buying me a coffee. I promise I won't spend it on beer instead... 😏